0025: Creating and Using a LinkButton
Today’s buttons are simple, a button to click to follow a link and another one with a pretty face and a link. So, what’s the difference?
A LinkButton
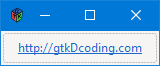
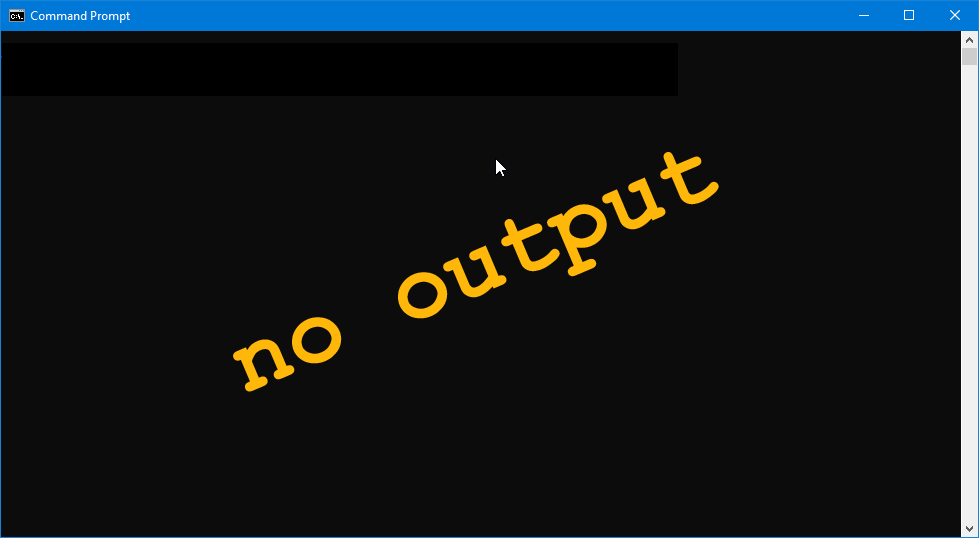
At its simplest, a LinkButton
has a link that takes the user to a website. And on its face, the LinkButton
shows the URL for that site.
And because this example is so simple, I’ve dumped it all into the TestRigWindow
object:
class TestRigWindow : MainWindow
{
string title = "LinkButton without Text";
string link = "http://gtkDcoding.com";
string message = "The text entry box holds: ";
LinkButton linkButton;
this()
{
super(title);
addOnDestroy(&endProgram);
linkButton = new LinkButton(link);
add(linkButton);
showAll();
} // this()
void endProgram(Widget w)
{
writeln(message, linkButton.getUri());
} // endProgram()
} // class TestRigWindow
Nothing to it, really, define the link, instantiate the LinkButton
object, add it, and the rest is taken care of for you. You won’t have to figure out anything else because it hooks into the OS to grab the default browser and passes it the URL.
But what if you don’t want the URL to show on the button? Perhaps your URL is something long and ugly or you want to take the user to a specific page that would end up looking like:
http://gtkdcoding.com/2019/01/11/0000-introduction-to-gtkDcoding.html
That ain’t gonna look so pretty on a button, so you might instead consider…
A LinkButton with a Pretty Face
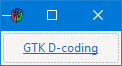
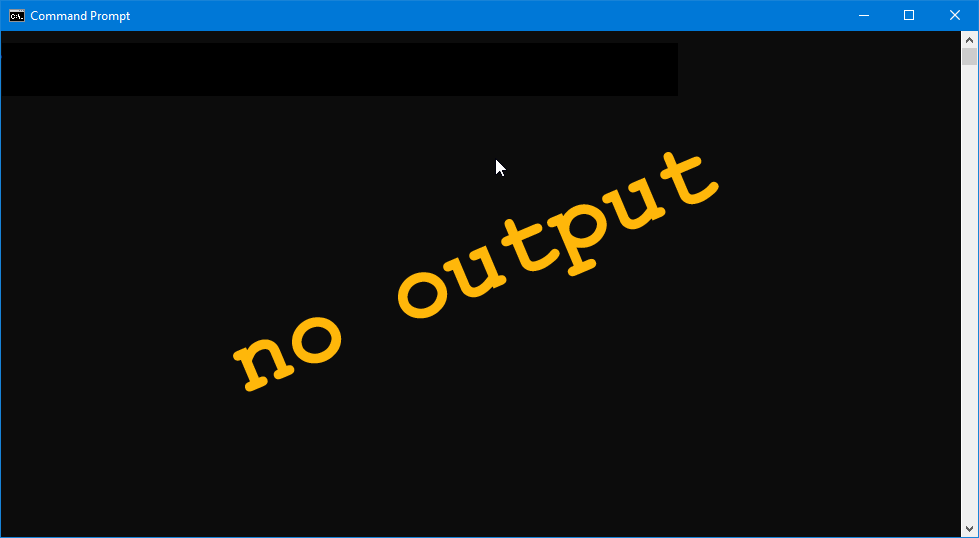
Not a lot changes:
class TestRigWindow : MainWindow
{
string title = "LinkButton with Text";
string link = "http://gtkdcoding.com/2019/01/11/0000-introduction-to-gtkDcoding.html";
string linkText = "GTK D-coding";
string message = "The text entry box holds: ";
LinkButton linkButton;
this()
{
super(title);
addOnDestroy(&endProgram);
linkButton = new LinkButton(link, linkText);
add(linkButton);
showAll();
} // this()
void endProgram(Widget w)
{
writeln(message, linkButton.getUri());
} // endProgram()
} // class TestRigWindow
It’s all in the arguments sent to LinkButton
’s constructor:
- Send it one argument, a URL, and it’s happy to slap that onto the face of the button.
- Send it two arguments, a URL and a nicely-formatted string, and that button ends up all cute and cuddly.
On the downside, the LinkButton
spits out a warning to the Command Prompt window every time you use it. My research has turned up no reasonable explanation and it’s been going on since the release of GTK 3.0. The good news is that the message is of no consequence and it seems safe to ignore.
And that’s it for this time, a short post for a short day. Come on back sometime and we’ll do this again.
Comments? Questions? Observations?
Did we miss a tidbit of information that would make this post even more informative? Let's talk about it in the comments.
- come on over to the D Language Forum and look for one of the gtkDcoding announcement posts,
- drop by the GtkD Forum,
- follow the link below to email me, or
- go to the gtkDcoding Facebook page.
You can also subscribe via RSS so you won't miss anything. Thank you very much for dropping by.
© Copyright 2024 Ron Tarrant