0090: Titlebar Icons
Today’s post is the result of special requests from GtkD Forum member Peter Pinkness and GreatSam4Sure over on the D Language Forum.
First up… Peter asked how to put an icon of his own choosing in the titlebar of a GTK application. And since we’re all gathered, we might as well discuss it.
And for the record, there are two ways to get a custom icon in the titlebar. Method number one is:
An Icon from an Image
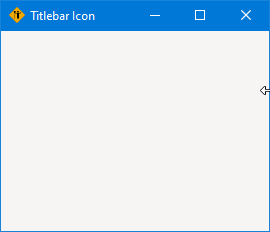
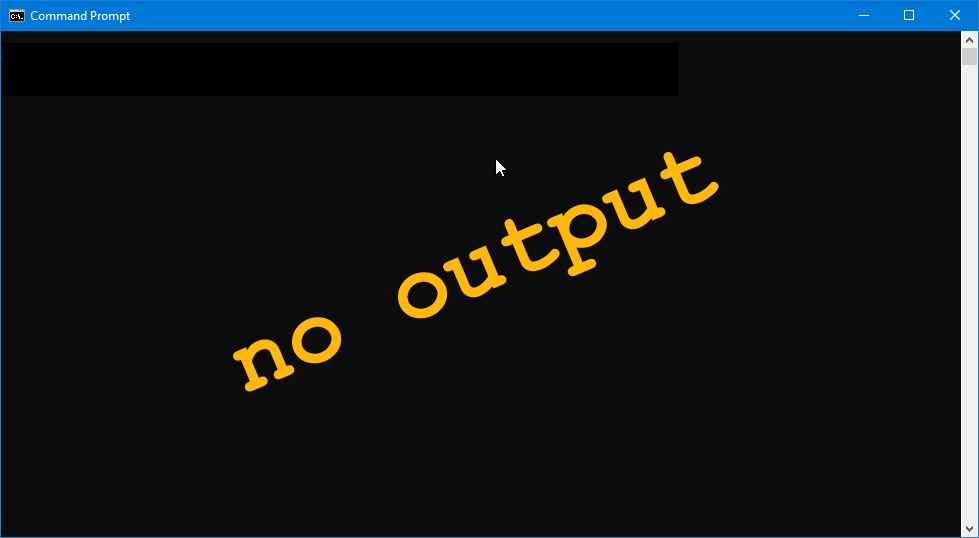
This first method entails loading an image directly into the titlebar’s icon. In context with the TestRigWindow
, it looks like this:
class TestRigWindow : MainWindow
{
string title = "Titlebar Icon";
AppBox appBox;
this()
{
super(title);
setSizeRequest(640, 480);
// load an image file to use an a titlebar icon
setIconFromFile("images/road_crew.png");
addOnDestroy(&quitApp);
appBox = new AppBox();
add(appBox);
showAll();
} // this()
void quitApp(Widget widget)
{
string exitMessage = "Bye.";
writeln(exitMessage);
Main.quit();
} // quitApp()
} // class TestRigWindow
One line of code is all it takes and boom, you’re done.
An Icon from a Pixbuf
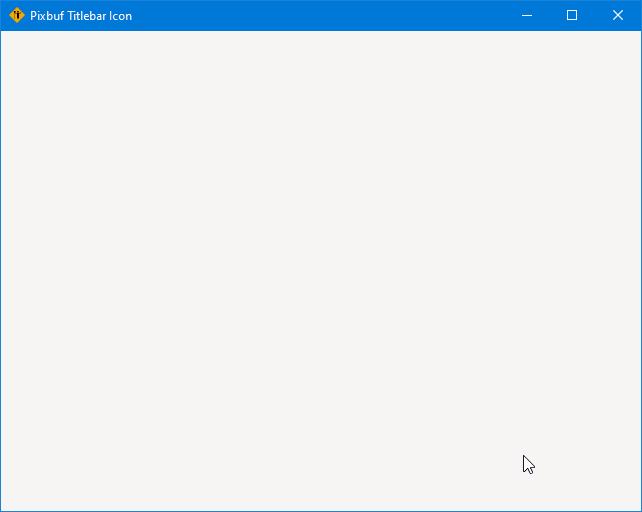
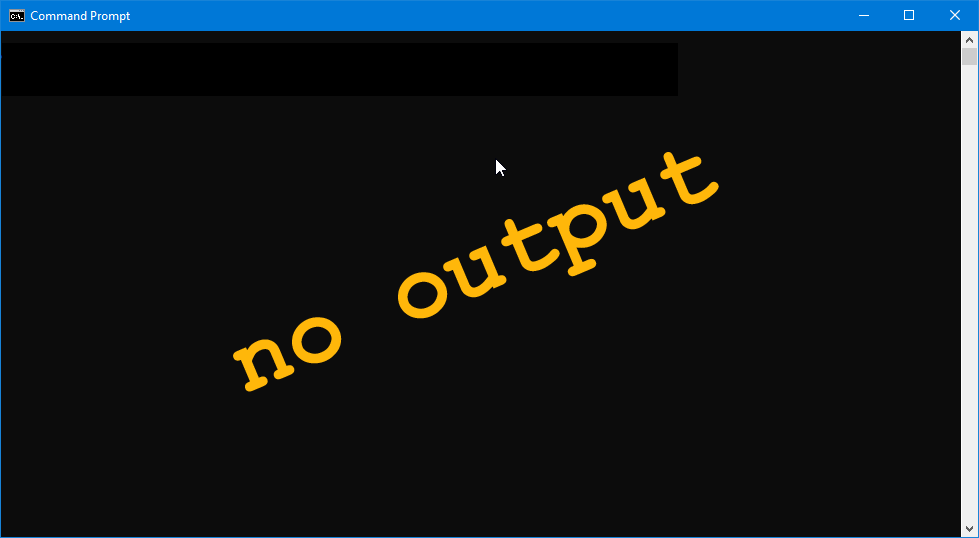
This method is twice as complicated as the first in that it takes two lines of code as seen here:
class TestRigWindow : MainWindow
{
string title = "Pixbuf Titlebar Icon";
AppBox appBox;
this()
{
super(title);
setSizeRequest(640, 480);
// alternate way to use a titlebar icon
Pixbuf pixbuf = new Pixbuf("images/road_crew.png");
setIcon(pixbuf);
addOnDestroy(&quitApp);
appBox = new AppBox();
add(appBox);
showAll();
} // this()
void quitApp(Widget widget)
{
string exitMessage = "Bye.";
writeln(exitMessage);
Main.quit();
} // quitApp()
} // class TestRigWindow
First, the Pixbuf
is created and filled from the same file we used in method one. Then, a call to setIcon()
and that’s it. Done like dinner.
Centering a Window
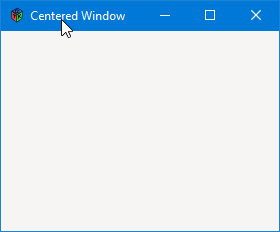
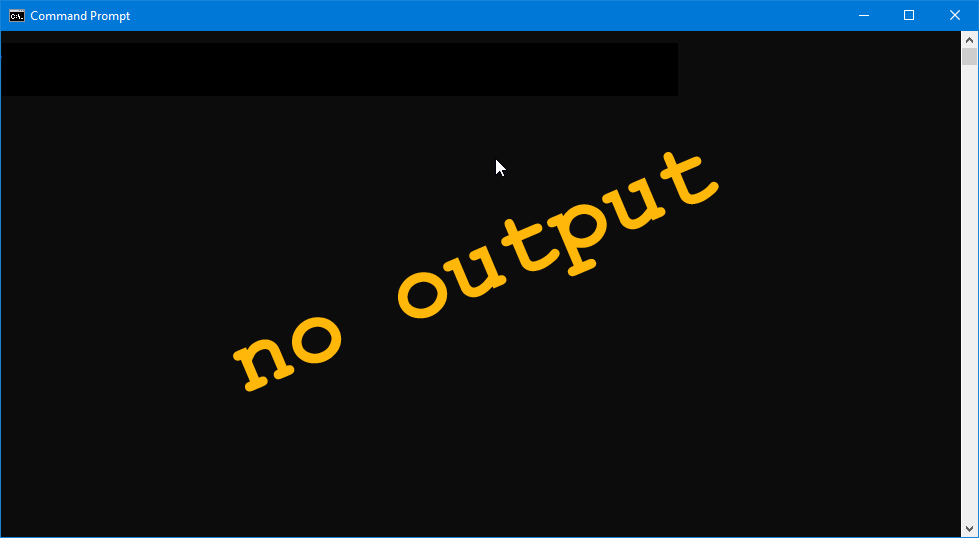
GreatSam4Sure asked how to center a window and it’s done like this:
class TestRigWindow : MainWindow
{
string title = "Centered Window";
AppBox appBox;
this()
{
super(title);
setPosition(WindowPosition.CENTER);
appBox = new AppBox();
add(appBox);
addOnDestroy(&quitApp);
showAll();
} // this()
void quitApp(Widget widget)
{
string exitMessage = "Bye.";
writeln(exitMessage);
Main.quit();
} // quitApp()
} // class TestRigWindow
In the constructor, after the call to the super-class constructor, a quick call to setPosition()
centers the window. The argument is an element of the WindowPosition enum
found on line 3921 of gtk/c/types.d.
And that’s all there is to that.
Conclusion
If you have any specific questions about how some aspect of GTK or its dependencies (GDK, Cairo, Pango) works, please feel free to email me using the link below. I’ll write it up, add it to the posting roster, and mention your name in the text. If you’d rather remain anonymous, please be sure to let me know.
Comments? Questions? Observations?
Did we miss a tidbit of information that would make this post even more informative? Let's talk about it in the comments.
- come on over to the D Language Forum and look for one of the gtkDcoding announcement posts,
- drop by the GtkD Forum,
- follow the link below to email me, or
- go to the gtkDcoding Facebook page.
You can also subscribe via RSS so you won't miss anything. Thank you very much for dropping by.
© Copyright 2023 Ron Tarrant