0113: Application IDs and Signals
Continuing from last time when we started looking at GTK/GIO Applications, today let’s look at…
Application IDs
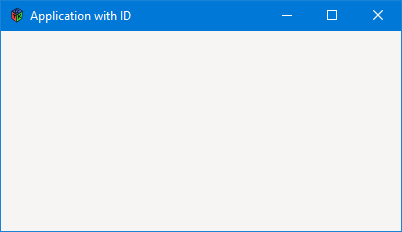
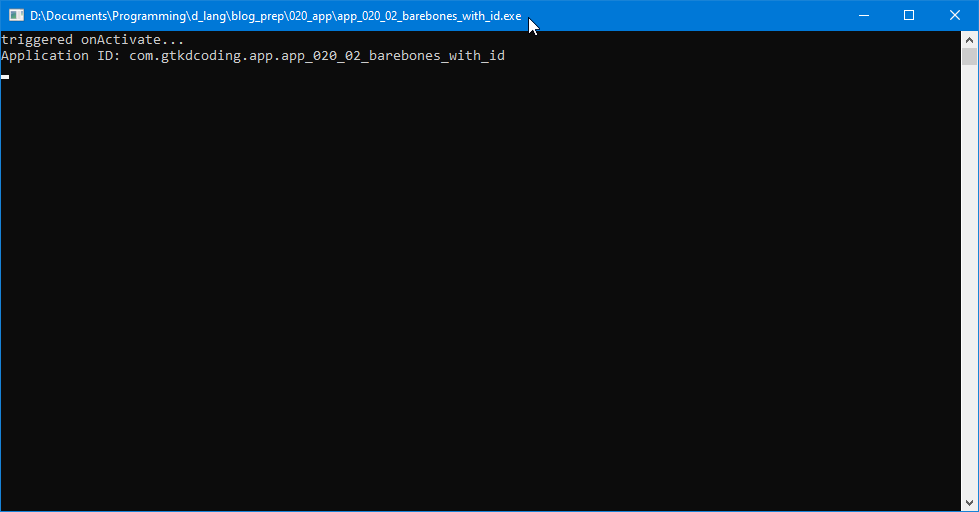
Every Application
has to have an ID
, even if—as in our example from last time—it’s null
. The intention is that each Application
can be singled out for inter-process communication, even across the vastness of the Internet. This may seem like overkill, but if you think about it, how else can we guarantee finding a single remote application among the (possibly) millions that may be accessible online? This also serves as a first line of defence against hacking that can be built into every Application
we write. It’s not a great defence, but while hackers are guessing the ID, they aren’t messing with our application.
You may wonder how we can be sure that the ID
we give our Application
hasn’t been used before by every Thomas, Richard, and Harold on the planet. The solution is rather ingenious and so simple, I wish I’d thought of it…
The ID
starts with a reverse-order URL. And if you don’t own a URL you can use an email account or the full URL of any online code repository you have read/write privileges for.
Then, we tack on a unique string identifier. And as long as we keep track of which identifiers we give to which application, there should never be a problem.
Here are the choices I had when naming the example used here, each based on a URL I have access to and the unique string is just the name of the code file:
- blog: com.gtkdcoding.app.app_02_barebones_with_id,
- email: com.gmail.gtkdcoding.app.app_02_barebones_with_id,
- repository: com.github.rontarrant.gtkdcoding.app.app_02_barebones_with_id
You’ll note that I added an extra layer (.app) between the URL and the file name. It’s not strictly necessary, but it’s part of the site organization, so I threw it in there. However, the directory where you’ll find this code file is named 020_app
, not app
. Why didn’t I use the full directory name?
Because we have…
Other Naming Conventions We Need to Follow
Interestingly enough, these conventions are similar to those used to name variables (as if we didn’t see that coming):
- the
ID
must be made up of at least two elements separated by a dot (element.element), - elements can be made up of:
- alpha-numeric characters (a-z/A-Z, 0-9),
- underscores (_), and
- hyphens (-),
- the first character in an
ID
cannot be:- a digit (0-9), or
- a dot (.),
- the first character in an element cannot be a digit (0-9),
- putting two dots together (..) is not allowed,
- the
ID
can’t start or end with a dot (.), and - the full length of the ID can’t be more than 255 characters.
So, naming the ID looks like this:
string id = "com.gtkdcoding.app.app_02_barebones_with_id"; // rules
Naming an ID
string can be patterned after a D-language import statement. We can simply use the file or project name and substitute a dot for a directory separator. And when it’s time to update the project to a new version, just toss in a version number… as long as it’s done in such a way that we don’t violate any of the naming conventions.
All this takes place in our derived class, MyApplication
(derived from GtkApplication
which, if you remember, is an alias of gtk.Application
and is, in turn, derived from gio.Application
).
Now, let’s move on to…
Application Signals
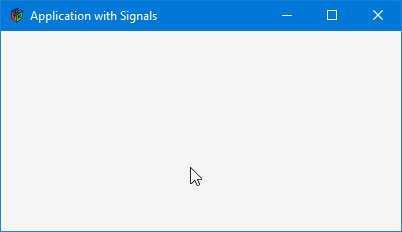
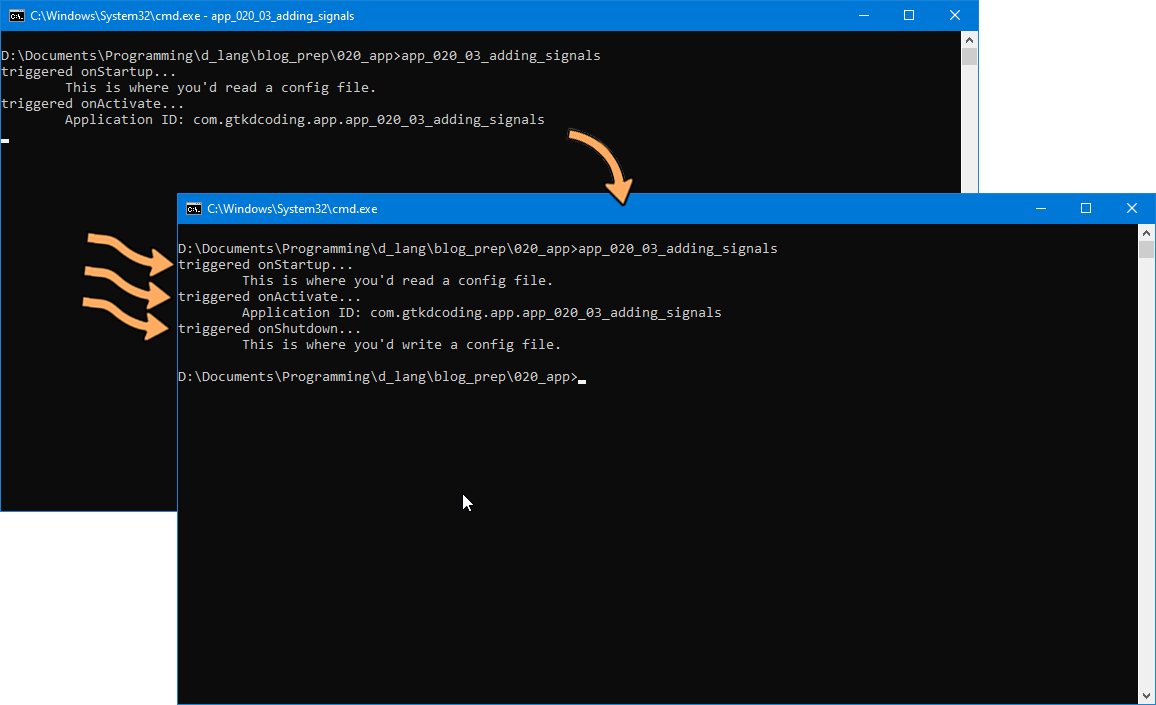
The two most obvious signals that every Application
might have are:
startup
, andshutdown
.
So much might be done to prepare for running an Application
or to clean up before exiting. A few things that come to mind are:
- loading and saving a configuration file,
- on shutdown, check for unsaved changes and see if the user wants to save before exiting, or
- on start-up, pop open a splash screen.
Hooking them up is no biggie. We just do the same thing we always do:
addOnStartup(&onStartup);
addOnShutdown(&onShutdown);
And the callback functions, in their simplest form, might look like:
void onStartup(GioApplication app) // non-advanced syntax
{
writeln("triggered onStartup...");
writeln("\tThis is where you'd read a config file.");
} // onStartup()
void onShutdown(GioApplication app) // non-advanced syntax
{
writeln("triggered onShutdown...");
writeln("\tThis is where you'd write a config file.");
} // onShutdown()
Conclusion
And that wraps it up for another day. Next time, we’ll tackle GIO/GTK flags. Until then—to paraphrase Les Nessman of WKRP, may the good code be yours.
Comments? Questions? Observations?
Did we miss a tidbit of information that would make this post even more informative? Let's talk about it in the comments.
- come on over to the D Language Forum and look for one of the gtkDcoding announcement posts,
- drop by the GtkD Forum,
- follow the link below to email me, or
- go to the gtkDcoding Facebook page.
You can also subscribe via RSS so you won't miss anything. Thank you very much for dropping by.
© Copyright 2024 Ron Tarrant