0110: Scale Button
Today we’re going to look at the Scale
button. Note that this is different from a ScaleButton
which we worked with in post #0047. Also, we won’t be stopping with just a simple demo. Instead, we’ll be using it as a springboard into a practical example that grew out of a request by Jan Hönig over on the DLang forum. Jan asked for a demo wherein a slider controls the position of a ball on a DrawingArea
. So today, we’ll start with this simple example of a plain-jane Scale
button and in the next blog post, we’ll go for the full-on demo Jan asked for.
The Scale Button
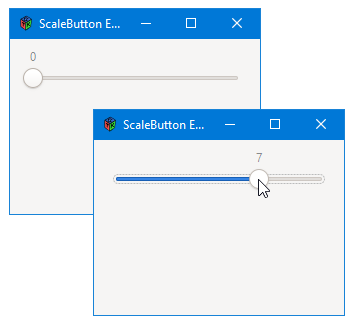
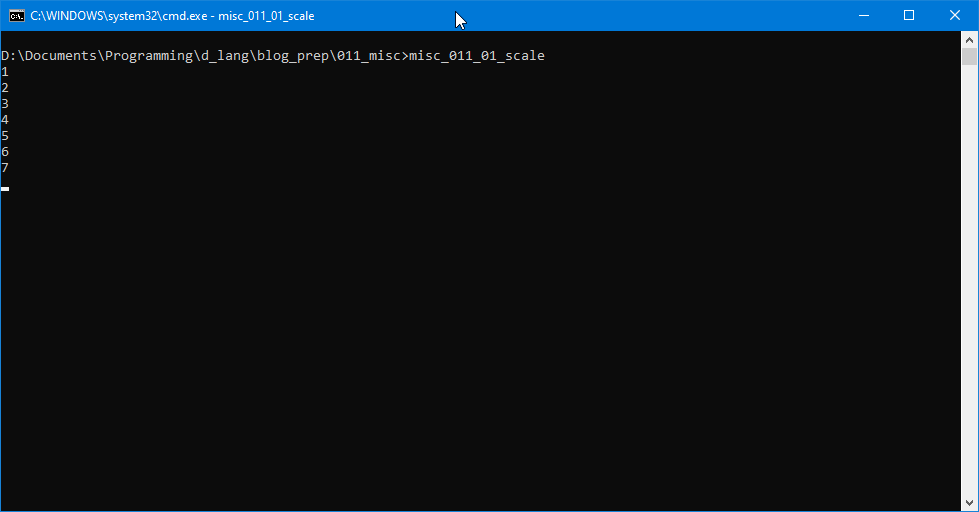
First things first. Since we haven’t yet talked about the Scale
button, let’s start by taking a look at the bare-bones version…
Imports
Right at the top, we have a couple of new import statements:
import gtk.Scale;
import gtk.Range;
Yup, the Scale
module is needed so we can work with the Scale
button. As to the Range
, we’ll talk about that when we get to the MyScale
class.
For now, here’s the `AppBox’:
The AppBox Stuff
Here’s the entire class (it’s very brief, so why not?):
class AppBox : Box
{
MyScale myScale;
int localPadding = 0, globalPadding = 10;
bool expand = false, fill = false;
this()
{
super(Orientation.VERTICAL, globalPadding);
myScale = new MyScale();
packStart(myScale, expand, fill, localPadding);
} // this()
} // class AppBox
All we’re doing here stuffing in an instance of a ‘MyScale’ class (derived from the Scale
). That’s it.
So, let’s talk about that…
The MyScale Class
Here’s the class:
class MyScale : Scale
{
double minimum = 0;
double maximum = 10;
double step = 1;
this()
{
super(Orientation.HORIZONTAL, minimum, maximum, step);
addOnValueChanged(&valueChanged);
} // this()
void valueChanged(Range range)
{
writeln(getValue());
} // valueChanged()
} // class MyScale
Right up front in the class preamble, we set up the details. And those details are what define the Range
I mentioned earlier:
minimum
,maximum
, andstep
.
The statement, addOnValueChanged(&valueChanged)
hooks up the Scale
button to its callback function. If you look at the valueChanged()
callback itself, you’ll see that the argument is a Range
. So, let’s talk about that…
Firstly, the Range
is internal, so don’t worry about figuring out how to access it. As the name implies, a Range
is a series of numbers with a minimum and a maximum (we just saw those in the preamble). They can be 0 to 10, -52 to +76… whatever you want.
We saw similar things before in the ScaleButton
demo, but as we’ve seen here, these two widgets are quite different. Don’t let the similarity in name throw you off.
Conclusion
That’s all we have time for today, so join us next time when we put this Scale
button to work in controlling the position of a simple graphic on a DrawingArea
.
Until then.
Comments? Questions? Observations?
Did we miss a tidbit of information that would make this post even more informative? Let's talk about it in the comments.
- come on over to the D Language Forum and look for one of the gtkDcoding announcement posts,
- drop by the GtkD Forum,
- follow the link below to email me, or
- go to the gtkDcoding Facebook page.
You can also subscribe via RSS so you won't miss anything. Thank you very much for dropping by.
© Copyright 2023 Ron Tarrant