0092: How Big is My Window
Last time, we were talking about placing a Window
and—if you remember—I also said I wanted my application to remember the Window
’s size as well, so let’s dig into that, shall we?
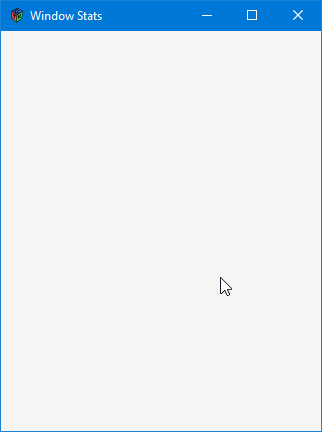
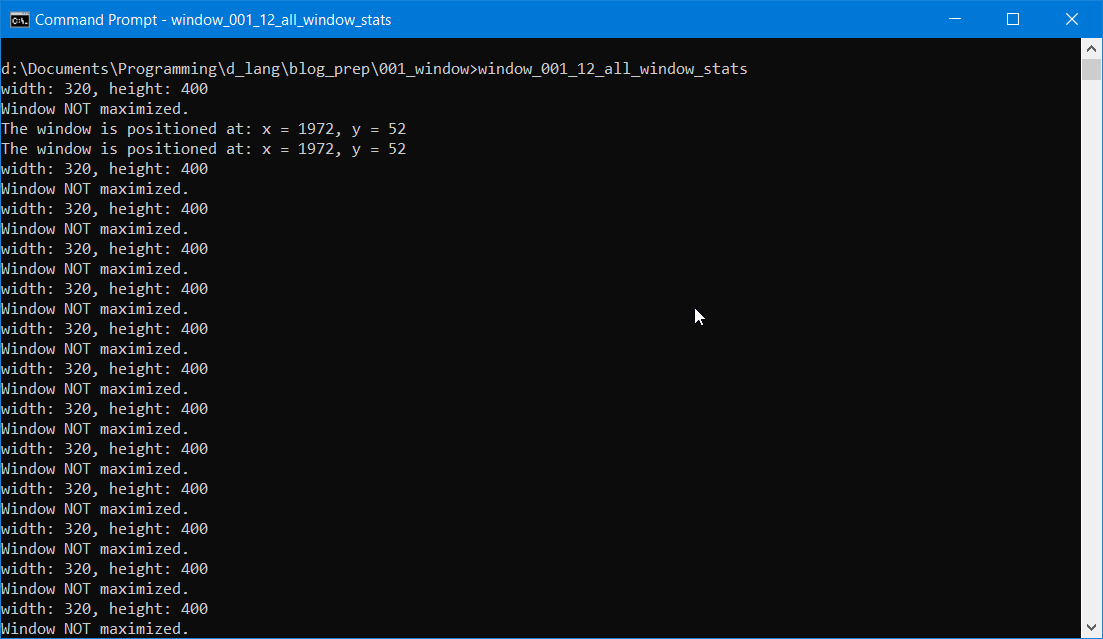
Another wrinkle we can toss in here is remembering whether the window is maximized or not when last it was used. This is something else that can be saved to a configuration file for user convenience.
An Addition to the Constructor
This time, we’ll hook up another signal as you can see here:
this()
{
super(title);
addOnDestroy(&quitApp);
addOnCheckResize(&onCheckResize); // NEW
addOnConfigure(&onConfigure);
appBox = new AppBox(this);
add(appBox);
showAll();
} // this()
Whereas the onConfigure
signal tells us when the window’s been moved, onCheckResize
tells us when the user resizes it. And this naturally needs a callback:
The onCheckResize Callback
And the callback takes a (perhaps) unexpected argument:
void onCheckResize(Container container)
{
getSize(width, height);
if(isMaximized)
{
_isMaximized = true;
}
else
{
_isMaximized = false;
}
writeln("width: ", width, ", height: ", height);
checkMaxState();
} // onCheckResize()
And what’s the purpose of the Container argument?
That’s the Widget
with which the check-resize signal is associated. In fact, when you look at the hierarchy of GTK Widget
s, the Window
class inherits from the Container
so we have all those Container
goodies in our Window
s, too.
Now, the main purpose of this callback is to keep us informed. In actual fact, other than setting our _isMaximized
flag, we don’t even need it… except for special circumstances, like, perhaps:
- if we want to force a redraw after the window is resized, or
- if we need to restructure the UI as the size of the
Window
changes.
However, both of those are dealt with automatically by GTK most of the time. Still, it’s good to know the mechanism is there if we need it.
We don’t even need it for gathering the new size stats because we can get that from Window.getSize()
which we could access whether the onCheckResize
signal fires or not.
What the onCheckResize
signal does do is give us a specific moment in the life cycle of our application when it feels natural to check the Window
state for whatever purpose suits us.
Other TestRigWindow Functions
We still have a couple of functions to look at, but neither needs much explanation:
showWindowStats()
has an extra statement to report theWindow
’swidth
andheight
, andcheckMaxState()
reports on the maximize state.
Both are stand-in functions as already explained and each would be replaced by functions for storing the Window
stats in a configuration file.
So, Why Pre-save Window Stats
It may cross your mind that, until the application is closing, we don’t need to gather all these Window
stats, but here’s the rub:
If we wait until the getDestroy
event, it’s too late. The Window is destroyed before the getDestroy()
callback is triggered, leaving us with no stats to gather.
And that’s why, in these circumstances, the configure-event
and check-resize
flags are so handy.
Conclusion
Next time, we’ll look at an alternate way to gather this data. Until then, may the great code be yours.
Comments? Questions? Observations?
Did we miss a tidbit of information that would make this post even more informative? Let's talk about it in the comments.
- come on over to the D Language Forum and look for one of the gtkDcoding announcement posts,
- drop by the GtkD Forum,
- follow the link below to email me, or
- go to the gtkDcoding Facebook page.
You can also subscribe via RSS so you won't miss anything. Thank you very much for dropping by.
© Copyright 2023 Ron Tarrant